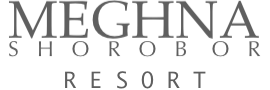
Sign up our Newsletter
Unlock a world of exclusive updates and captivating content by subscribing to our newsletter!
To add Toastr notifications in a Laravel application, you can follow these steps:
Step 1: Install Laravel If you haven't already, make sure you have Laravel installed and set up. You can install Laravel using Composer:
composer create-project --prefer-dist laravel/laravel your-project-name
Step 2: Install Toastr via Composer You can use the "toastr" package, which is a popular JavaScript library for displaying notifications.
composer require brian2694/laravel-toastr
Step 3: Configure Toastr After installation, you need to configure Toastr in your Laravel application.
config/app.php
file.'providers'
array, add the following line to register the service provider:Brian2694\Toastr\ToastrServiceProvider::class,
'aliases'
array, add the following line to register the Toastr facade:'Toastr' => Brian2694\Toastr\Facades\Toastr::class,
Step 4: Publish Configuration and Assets You need to publish the Toastr configuration file and assets to customize the notifications.
php artisan vendor:publish --provider="Brian2694\Toastr\ToastrServiceProvider"
toastr.php
file in the config
directory of your Laravel application. You can customize notification settings in this file.Step 5: Add Toastr JavaScript and CSS To include the Toastr JavaScript and CSS files in your application, you can add them to your layout file.
resources/views/layouts
directory), include the JavaScript and CSS files:<link rel="stylesheet"href="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/css/toastr.min.css"><script src="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/js/toastr.min.js"></script>
Step 6: Display Toastr Notifications Now, you can use Toastr to display notifications in your Laravel application. Here's an example of how to use it in a controller or a route:
use Toastr; public function someFunction() { // Your code here Toastr::success('Your success message', 'Success'); return redirect()->route('your.route.name'); }
You can use various Toastr methods, such as success
, info
, warning
, and error
, to display different types of notifications.
Step 7: Show Toastr Notifications in Your View In your main layout file (usually in the resources/views/layouts
directory), include the following line to display the notifications:
{!! Toastr::render() !!}
This line will render and display the notifications at the appropriate location on your web page.
With these steps, you can integrate Toastr notifications into your Laravel application to provide user-friendly and customizable notification messages. Make sure to refer to the Toastr documentation for more customization options and advanced usage.